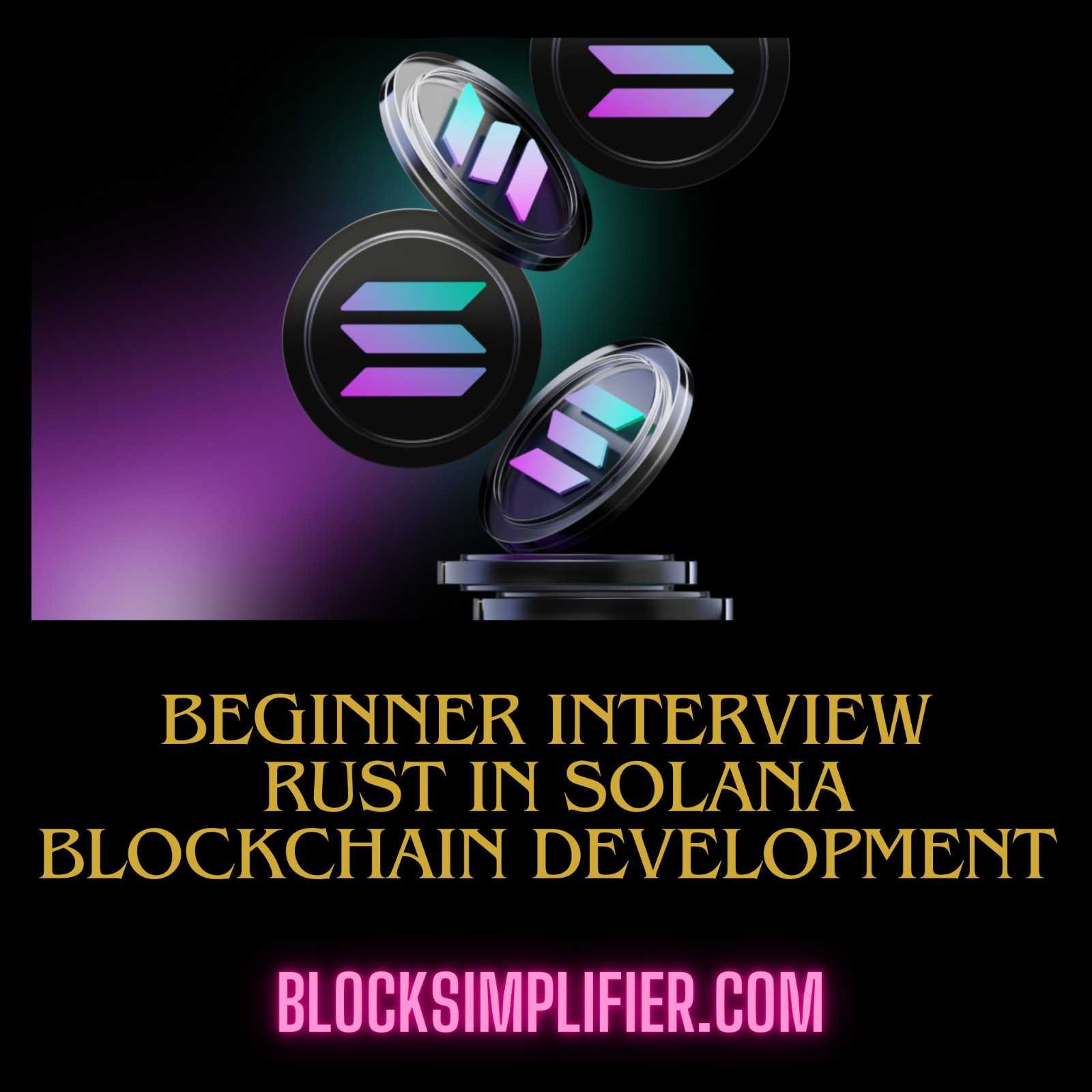
Here are Most asking questions with answers on Rust in Solana Blockchain Development
Table of Contents
Basics of Solana and Rust
- What is Solana?
Solana is a excessive-overall performance blockchain known for its speedy transaction speeds and coffee fees, the usage of Proof of History (PoH) for scalability. - Why use Rust for Solana clever contracts? Rust is memory-safe, speedy, and stops commonplace security troubles, making it ideal for blockchain development.
- What is a Solana Program?
A Solana Program is a clever agreement deployed at the Solana blockchain, written in Rust or C. - How does Solana differ from Ethereum?
Solana makes use of PoH and parallel transaction execution, while Ethereum makes use of Proof of Stake (PoS) and serial execution. - How do you install Rust for Solana development?
Install Rust usingcurl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- What is the Solana CLI?
It is a command-line tool to interact with the Solana blockchain. Install it usingsh -c "$(curl -sSfL https://release.solana.com/stable/install)"
- How do you create a new Solana program?
cargo install --git https://github.com/solana-labs/anchor anchor-cli anchor init my_program
- What is Anchor in Solana?
Anchor is a Rust framework that simplifies Solana smart contract development with predefined macros and utilities. - What is the default programming model in Solana?
Solana follows an account-based model rather than an Ethereum-like state machine. - How do Solana accounts work?
Accounts store data and program state, acting as persistent storage for Solana programs.
Rust Programming in Solana
- What Rust dependencies are needed for Solana smart contracts?
solana-program
borsh
anchor-lang
anchor-spl
- What is the entry point of a Solana program?
entrypoint!(process_instruction);
- How do you serialize and deserialize data in Solana programs?
Use Borsh for efficient serialization:#[derive(BorshSerialize, BorshDeserialize)] pub struct MyData { pub count: u64, }
- How do you define a Solana instruction?
#[derive(AnchorSerialize, AnchorDeserialize)] pub struct MyInstruction { pub amount: u64, }
- How do you handle accounts in Solana?
#[account(mut)] pub my_account: Account<'info, MyData>,
- What is the difference between
ProgramAccount
andUncheckedAccount
?ProgramAccount
is a safe wrapper for deserialized accounts, whileUncheckedAccount
is a raw Solana account. - How do you implement a Solana program?
#[program] pub mod my_program { use super::*; pub fn initialize(ctx: Context<Initialize>) -> ProgramResult { let my_account = &mut ctx.accounts.my_account; my_account.count = 0; Ok(()) } }
- What is
Context
in Anchor?Context<T>
provides access to accounts and program data. - How do you log messages in Solana smart contracts?
msg!("Transaction executed successfully!");
- What is
AccountInfo
in Solana?
It is a struct that provides metadata about a Solana account, including its owner and lamports.
Solana Transactions & Accounts
- What are lamports?
The smallest unit of SOL (1 SOL = 1 billion lamports). - How do you send SOL in a Solana program?
invoke( &system_instruction::transfer(&from.key, &to.key, amount), &[from.clone(), to.clone(), system_program.clone()], )
- How do you check the balance of an account?
solana balance <ACCOUNT_ADDRESS>
- How do you create a PDA (Program Derived Address)?
let pda = Pubkey::create_program_address(&[b"seed"], program_id)?;
- What is CPI (Cross-Program Invocation) in Solana?
Calling one Solana program from another. - How do you call another Solana program from within a program?
invoke_signed( &instruction, &[account.clone()], &[&[b"seed", &[bump]]], )
- What is the difference between
invoke
andinvoke_signed
?invoke_signed
allows the use of PDAs with signatures. - How do you sign a transaction in Solana?
solana transaction sign --signer <keypair.json>
- How do you deploy a Solana program?
solana program deploy target/deploy/my_program.so
- How do you check the logs of a Solana program?
solana logs
Solana Anchor Framework
- What is
#[account]
in Anchor?
A macro that defines account structures. - How do you initialize an account using Anchor?
#[derive(Accounts)] pub struct Initialize<'info> { #[account(init, payer = user, space = 8 + 8)] pub my_account: Account<'info, MyData>, pub user: Signer<'info>, }
- What does
#[instruction(...)]
do in Anchor?
Passes extra parameters into the program. - What is the purpose of
#[derive(Accounts)]
?
It simplifies account validation. - How do you generate an IDL (Interface Definition Language) for a Solana program?
anchor build
Testing & Debugging
- How do you test Solana smart contracts?
anchor test
- What testing framework is used in Solana?
Mocha with JavaScript or Rust’s built-in test framework. - How do you simulate transactions locally?
solana-test-validator
Advanced Topics
- How do you upgrade a Solana program?
solana program deploy --upgrade
- What is a Token Account in Solana?
A special account used for holding SPL tokens. - How do you mint an SPL token?
spl-token mint <TOKEN_ADDRESS> <AMOUNT>
Security in Solana Smart Contracts
- What are common security risks in Solana smart contracts?
- Missing account validation
- Unchecked arithmetic overflows
- Privilege escalation attacks
- Unvalidated cross-program invocations
- How do you prevent reentrancy attacks in Solana?
Solana transactions are atomic, preventing external reentrancy. However, ensure proper account validation and program flow. - What is an unchecked account in Solana?
UncheckedAccount
allows access to any account but does not enforce ownership or data validation. - How do you ensure only the expected user modifies an account?
if ctx.accounts.my_account.owner != ctx.accounts.signer.key {
return Err(ProgramError::IncorrectProgramId);
}
- How do you verify the signer of a transaction?
if !ctx.accounts.signer.is_signer {
return Err(ProgramError::MissingRequiredSignature);
}
- How do you securely transfer tokens in Solana?
Use thespl-token
program with explicit authorization checks before transfers. - How can you prevent integer overflows in Solana programs?
Use Rust’schecked_add()
,checked_sub()
, andchecked_mul()
instead of direct arithmetic. - What is
require!
in Anchor?
A macro to enforce conditions:
require!(amount > 0, ErrorCode::InvalidAmount);
- How do you validate a PDA (Program Derived Address) before using it?
let (expected_pda, _) = Pubkey::find_program_address(&[b"seed"], program_id);
require!(pda.key == expected_pda, ErrorCode::InvalidPDA);
- How do you restrict instruction execution to the program owner?
Validate the program ID before processing logic. - How do you ensure that an instruction modifies an account only if necessary?
UseAccountInfo::is_writable
to check before modifying. - How do you prevent unauthorized CPI (Cross-Program Invocations)?
Validate accounts before executinginvoke
orinvoke_signed
.
DeFi on Solana
- What is an AMM (Automated Market Maker) on Solana?
A decentralized protocol that facilitates trading via liquidity pools (e.g., Raydium, Serum). - How do you interact with an AMM using Rust?
Use theserum_dex
orraydium
crate for programmatic trading. - What is a Solana lending protocol?
A protocol like Solend or Jet that enables lending and borrowing of assets. - How do you create a DeFi vault on Solana?
- Define a vault account
- Store deposited funds
- Implement a withdrawal function with ownership validation
- How do you interact with the Solana Token Program?
let mint = ctx.accounts.token_mint.key();
let authority = ctx.accounts.user.key();
let amount = 100;
let cpi_accounts = Transfer {
from: ctx.accounts.source.to_account_info(),
to: ctx.accounts.destination.to_account_info(),
authority: ctx.accounts.owner.to_account_info(),
};
- How do you calculate swap rates in a Solana AMM?
Use the constant product formula:
x * y = k
where x
and y
are reserves of two assets, and k
is a constant.
- How do you create a staking smart contract in Solana?
- Store staked token balance
- Apply time-based rewards
- Ensure proper withdrawals
- How do you prevent flash loan attacks in Solana DeFi protocols?
- Use time-based lockups
- Verify collateralization ratios
- Implement withdrawal limits
NFT Development on Solana
- What is an NFT on Solana?
A unique, non-fungible token following the Metaplex standard. - What is Metaplex?
A framework for creating, managing, and selling NFTs on Solana. - How do you mint an NFT on Solana?
metaplex upload assets/
metaplex create_candy_machine
- How do you create an NFT metadata account?
let metadata = Metadata {
name: "MyNFT".to_string(),
symbol: "NFT".to_string(),
uri: "https://example.com/metadata.json".to_string(),
};
- What is a Candy Machine?
A Metaplex protocol that automates NFT minting. - How do you transfer an NFT in Solana?
Use thespl-token
transfer instruction. - What are royalties in Solana NFTs?
Fees paid to the original creator on resale, enforced by the Metaplex standard. - How do you list an NFT for sale on a Solana marketplace?
- Use Metaplex auctions
- Interact with the Solana Program Library (SPL)
- How do you verify NFT ownership in Solana?
Check the token account’s metadata. - How do you create a fractionalized NFT on Solana?
- Create SPL tokens representing fractional ownership.
Solana Validator Setup
- What is a Solana Validator?
A node that validates transactions and secures the network. - How do you set up a Solana validator?
solana-install init
solana-validator --identity validator-keypair.json
- How do you generate a validator keypair?
solana-keygen new --outfile validator-keypair.json
- How do you check the current Solana epoch?
solana epoch-info
- How do you stake SOL as a validator?
solana create-stake-account stake-keypair.json 1000
- How do you monitor validator performance?
solana-validator-monitor
- How much SOL is required to run a validator?
The amount varies, but 10 SOL+ is recommended for transaction fees. - How do you vote as a validator?
Validators must create a vote account and submit votes to confirm transactions. - How do you update a Solana validator?
solana-install update
- What is a delinquent validator in Solana?
A validator that fails to produce blocks for several epochs. - How do you exit validator mode?
solana-validator exit