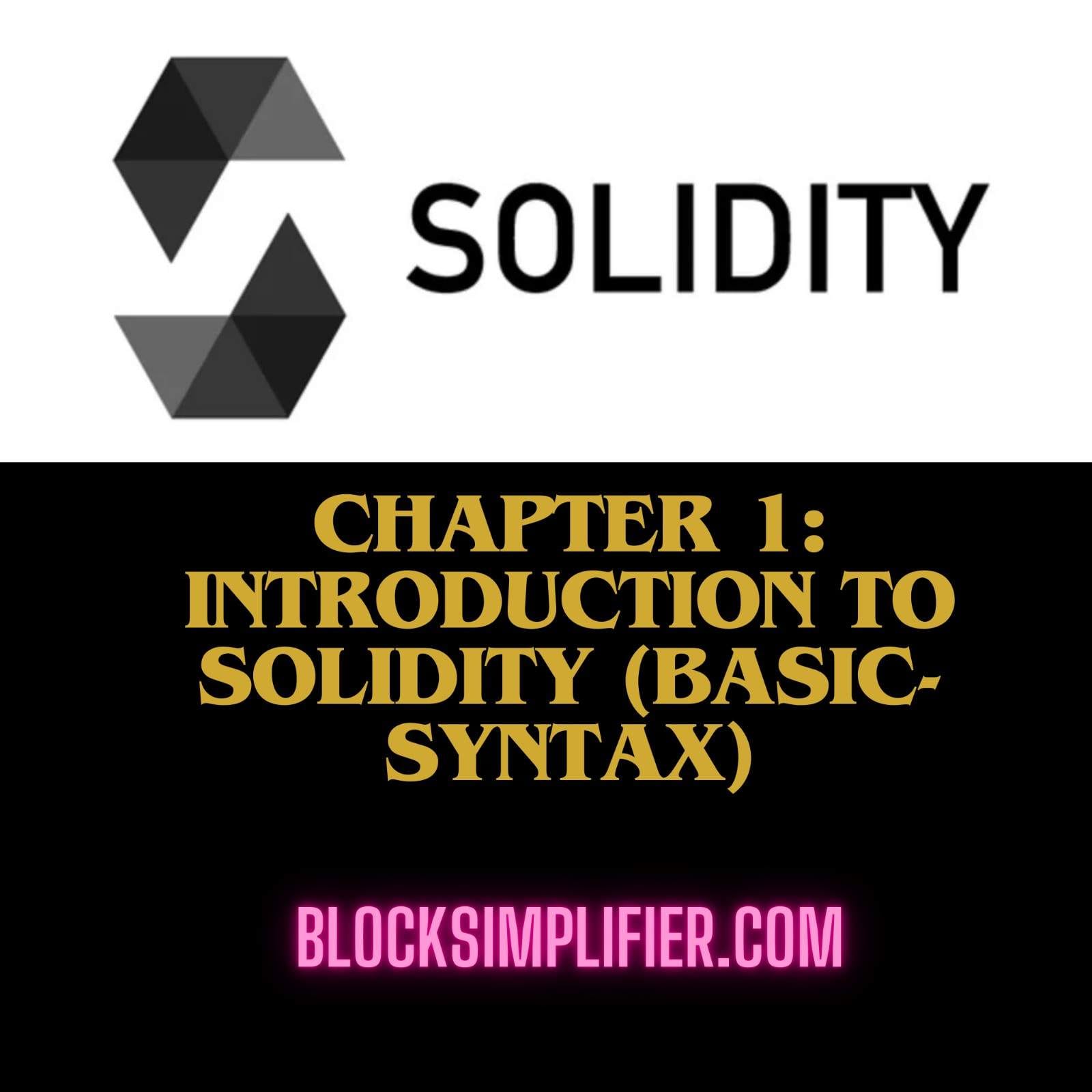
Solidity is a excessive-stage, statically typed programming language specially designed for writing clever contracts on blockchain structures. Developed with the Ethereum Virtual Machine (EVM) in mind, Solidity permits developers to create decentralized applications (DApps) that execute programmable common experience at the blockchain.. Its syntax attracts notion from famous languages like JavaScript, Python, and C , making it available to developers familiar with those languages.
Smart contracts written in Solidity are self-executing programs that facilitate, verify, and implement the negotiation or execution of a digital agreement with out intermediaries. These contracts can govern virtual asset transactions, automate enterprise common sense, and allow decentralized governance.
Table of Contents
History of Solidity
Solidity’s journey started in 2014 while Gavin Wood, one of Ethereum’s co-founders, brought it as the primary language for growing clever contracts at the Ethereum blockchain. The language became designed to provide a developer-pleasant environment for developing strong and steady applications.
- Initial Development (2014): Solidity turned into advanced via Christian Reitwiessner, Gavin Wood, and other center participants to the Ethereum undertaking.
- Mainstream Adoption (2015): With the launch of Ethereum’s mainnet, Solidity have become the de facto standard for clever agreement development.
- Feature Enhancements (2017-2019): The creation of advanced features consisting of libraries, inheritance, and modifiers improved the language’s flexibility and security.
- Compiler Updates: Regular updates to the Solidity compiler (solc) introduced better errors managing, optimization techniques, and static evaluation equipment to decorate the developer experience.
- Identity Management: Decentralized identification answers make use of Solidity to create verifiable credentials and identity systems.
Applications of Solidity in Blockchain Development
Solidity’s versatility has led to its adoption in a wide range of blockchain applications:
- Decentralized Finance (DeFi): Solidity powers DeFi platforms like Uniswap, Compound, and Aave, enabling decentralized trading, lending, and borrowing.
- Non-Fungible Tokens (NFTs): Smart contracts written in Solidity form the backbone of NFT marketplaces and platforms like OpenSea and Rarible.
- Decentralized Autonomous Organizations (DAOs): Solidity facilitates the creation of DAOs, allowing communities to manage shared resources and decision-making processes transparently.
- Gaming and Metaverse: Blockchain games like Axie Infinity and metaverse projects like Decentraland leverage Solidity for asset ownership and in-game economies.
- Supply Chain Management: Solidity-based smart contracts enhance transparency and traceability in supply chains.
- Identity Management: Decentralized identity solutions utilize Solidity to create verifiable credentials and identity systems.
Tools and Frameworks for Solidity Development
A robust ecosystem of tools and frameworks supports Solidity developers in building, testing, and deploying smart contracts efficiently:
- Compilers:
- Solc: The native Solidity compiler.
- Hardhat Solidity Compiler: Integrated with the Hardhat development environment for streamlined workflows.
- Development Frameworks:
- Hardhat: A flexible development surroundings for compiling, checking out, and deploying smart contracts.
- Truffle: A suite of gear for developing and coping with Ethereum-primarily based packages.
- Foundry: A subsequent-era framework focused on speed and developer productivity.
- Testing Tools:
- Chai and Mocha: JavaScript libraries used for writing and running contract tests.
- Ethers.js and Web3.js: Libraries for interacting with Ethereum contracts in tests and applications.
- Security Analysis Tools:
- MythX: A security analysis platform for identifying vulnerabilities in Solidity code.
- Slither: A static analysis framework to detect bugs and security issues.
- Certora: Formal verification tools for proving the correctness of smart contracts.
- Blockchain Interactions:
- Remix IDE: A web-based integrated development environment for writing and deploying Solidity contracts.
- Ganache: A personal blockchain for rapid testing and debugging.
- Deployment Tools:
- Infura: A service for interacting with the Ethereum blockchain without running a full node.
- Alchemy: A developer platform providing APIs for blockchain development.
Key Features of Solidity:
- High-Level Syntax: Easy-to-understand syntax inspired by popular programming languages.
- Statically Typed: Variables and types must be declared explicitly, ensuring fewer runtime errors.
- Object-Oriented: Supports inheritance, libraries, and complex user-defined types.
- Access Modifiers: Control visibility and accessibility of contract functions and variables.
- Event-Driven: Emit events to log important activities or states, allowing off-chain monitoring.
- Upgradeable Contracts: Can implement upgradeable and modular smart contract architectures.
- Supports Standards: Facilitates ERC standards like ERC-20, ERC-721, and ERC-1155.
Why Solidity Matters:
- It is the most widely used language for developing blockchain applications.
- Provides gear for creating decentralized systems that do away with the want for intermediaries.
- Enables the creation of programmable assets and agreements.
Solidity – Basic Syntax
The syntax of Solidity is straightforward, in particular for builders acquainted with languages like JavaScript. Let’s discover the foundational elements of Solidity programming.
1. Pragma Directive
Every Solidity report starts offevolved with a pragma directive, which specifies the compiler model to make sure compatibility.
pragma solidity ^0.9.0;
The ^0.9.0
indicates compatibility with any compiler version from 0.8.0 up to
2. Contract Declaration
A contract is the essential building block in Solidity, just like a class in item-orientated programming.
pragma solidity ^0.8.0;
contract MyContract {
// Code for the smart contract goes here
}
3. State Variables
State variables store data permanently on the blockchain.
contract Example {
uint256 public count = 0; // Unsigned integer variable
string public name = "Solidity"; // String variable
bool public isActive = true; // Boolean variable
}
4. Functions
Functions in Solidity contain the logic of your smart contract. They can be public
, private
, internal
, or external
.
Example of a Simple Function:
contract Example {
uint256 public count = 0;
// Function to increment the count
function increment() public {
count += 1;
}
}
5. Data Types
Solidity supports several data types:
- Integer:
uint
(unsigned) andint
(signed) of various sizes (uint8
,uint16
, etc.). - Boolean:
bool
type with valuestrue
orfalse
. - String:
string
type for text data. - Address: A unique 20-byte value that represents Ethereum addresses.
- Bytes: Fixed-size (
bytes1
tobytes32
) or dynamically sized (bytes
) byte arrays.
6. Control Structures
Solidity supports conditional statements (if
, else
), loops (for
, while
), and error handling (require
, assert
).
Example of Conditional Statement:
contract Example {
function isEven(uint256 _num) public pure returns (bool) {
if (_num % 2 == 0) {
return true;
} else {
return false;
}
}
}
7. Payable Functions
Payable functions enable contracts to receive Ether.
contract PayableExample {
address public owner;
constructor() {
owner = msg.sender; // Set contract deployer as owner
}
// Payable function to receive Ether
function deposit() public payable {}
// Check contract balance
function getBalance() public view returns (uint256) {
return address(this).balance;
}
}
Frequently Asked Questions (FAQ)
What is Solidity used for?
Solidity is used for writing smart contracts on blockchain platforms like Ethereum. These contracts enable the creation of decentralized applications, including DeFi platforms, NFT marketplaces, and DAOs.
Is Solidity hard to study?
Solidity’s syntax is just like JavaScript, making it extraordinarily smooth for developers with programming enjoy to study. However, gaining knowledge of blockchain-precise ideas requires extra effort.
Can Solidity be used outside Ethereum?
Yes, Solidity can be used on other EVM-compatible blockchains, such as Binance Smart Chain, Polygon, and Avalanche.
What gear are essential for Solidity improvement?
Key tools include Remix IDE for coding and deploying, Hardhat or Truffle for testing and managing projects, and security analysis tools like MythX and Slither.
How does Solidity handle security?
Solidity includes features like modifiers, custom error messages, and libraries to enhance security. Developers must also perform thorough audits and use static analysis tools to mitigate vulnerabilities.
2 thoughts on “Chapter 1: Introduction to Solidity (Basic-Syntax)”