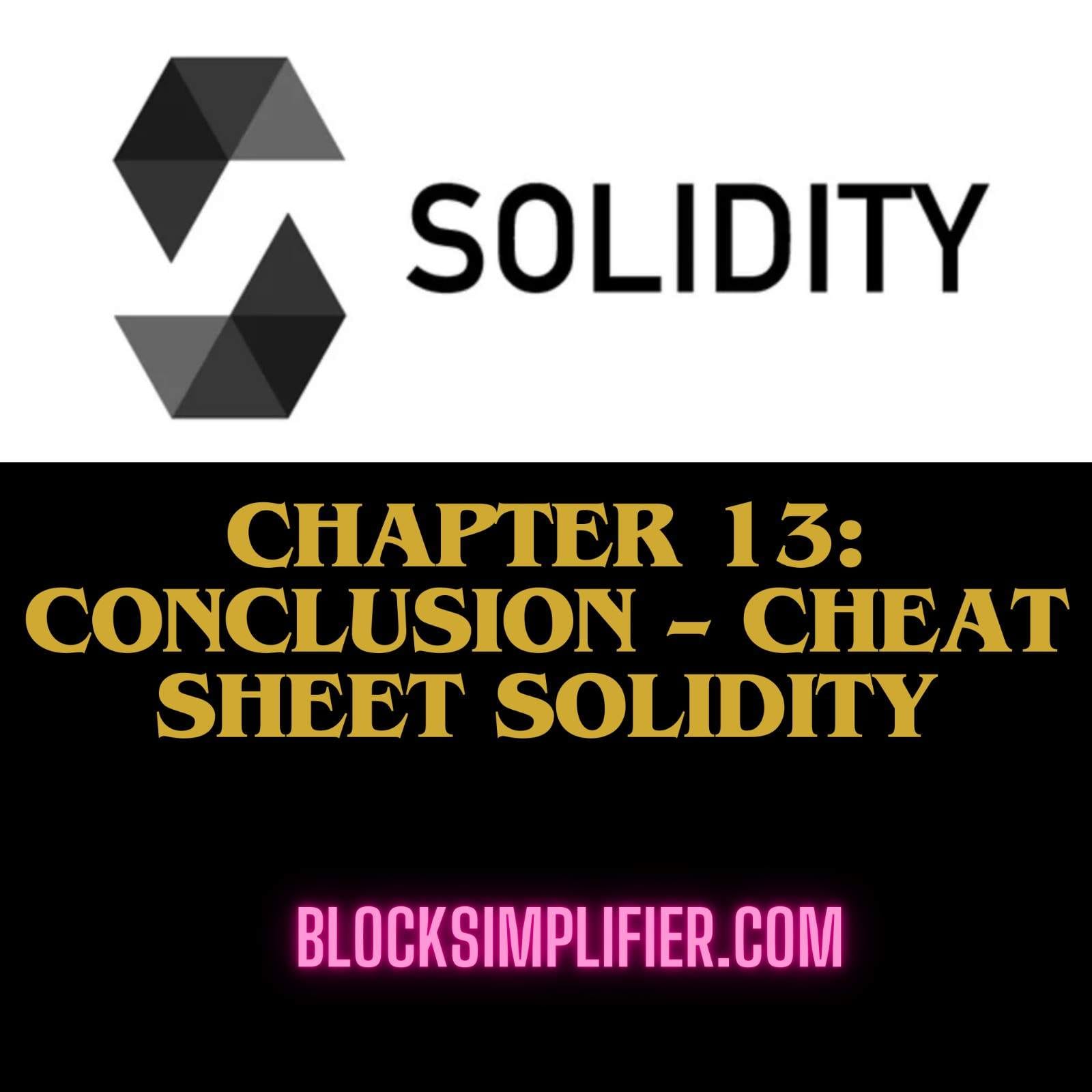
Master Solidity with our Cheat Sheet by BlockSimplifier! Simplify smart contract development with key syntax, tips, and best practices for Ethereum and blockchain programming.
Table of Contents
Recap of Key Learnings
1. Basic Structure of a Contract
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract MyContract { // State variables, functions, events, etc. }
Important Keywords:
pragma solidity
: Specifies the version of Solidity used.contract
: Defines a new contract.constructor
: A special function executed once upon contract creation.modifier
: Functions that can alter the behavior of other functions.event
: Used to log information that can be captured outside the contract.
2. State Variables
uint256 public balance; address public owner;
Public State Variables: Automatically create a getter function.
Private State Variables: Accessible only within the contract.
3. Constructor
constructor(uint256 _initialBalance) { balance = _initialBalance; owner = msg.sender; // Sets the deployer as the owner }
4. Functions
Function Declaration
function setBalance(uint256 _balance) public { balance = _balance; }
public
: Accessible both externally and internally.internal
: Accessible only within the contract or derived contracts.private
: Accessible only within the contract.view
: Function that doesn’t modify state, only reads.pure
: Function that neither modifies nor reads the state.
Example of a View Function
function getBalance() public view returns (uint256) { return balance; }
Example of a Pure Function
function multiply(uint256 a, uint256 b) public pure returns (uint256) { return a * b; }
5. Modifiers
modifier onlyOwner() { require(msg.sender == owner, "Not the owner"); _; } function changeOwner(address newOwner) public onlyOwner { owner = newOwner; }
require(condition, message)
: Throws an error if the condition is false.
_;
: Placeholder for where the function body will be inserted.
6. Events
event Transfer(address indexed from, address indexed to, uint256 amount); function transfer(address _to, uint256 _amount) public { emit Transfer(msg.sender, _to, _amount); }
Indexed parameters: Makes them searchable in the transaction logs.
7. Error Handling
require(balance >= _amount, "Insufficient balance"); revert("Something went wrong"); assert(x == 5);
require(condition, message)
: Ensures a condition is true.revert(message)
: Reverts the transaction with an optional message.assert(condition)
: Used for checking invariants, and will consume all gas if failed.
8. Payable Functions
function deposit() public payable { balance += msg.value; }
msg.value
: Returns the amount of Ether sent with the transaction.
9. Accessing the Blockchain Data
msg.sender
: The address that called the function.msg.value
: The amount of Ether sent with the call.block.timestamp
: The current block’s timestamp.block.number
: The current block’s number.tx.origin
: The address that initiated the transaction.
10. Storage vs Memory vs Stack
- Storage: Variables stored on the blockchain (persistent).
- Memory: Temporary data that only exists during execution.
- Stack: A very small, temporary space for local variables.
11. Inheritance
contract Parent { uint256 public x = 10; } contract Child is Parent { function getX() public view returns (uint256) { return x; // Inherited from Parent } }
12. Interfaces
interface IERC20 { function transfer(address recipient, uint256 amount) external returns (bool); } contract TokenInteraction { IERC20 token; function setTokenAddress(address _tokenAddress) public { token = IERC20(_tokenAddress); } function transferTokens(address recipient, uint256 amount) public { token.transfer(recipient, amount); } }
13. Libraries
library Math { function add(uint256 a, uint256 b) public pure returns (uint256) { return a + b; } } contract TestLibrary { using Math for uint256; function testAddition(uint256 a, uint256 b) public pure returns (uint256) { return a.add(b); // Calls the Math library } }
Selfdestruct
function closeContract() public onlyOwner { selfdestruct(payable(owner)); }
Gas Optimization Tips
- Use
uint256
instead of smaller types for consistent gas costs. - Minimize state changes to reduce gas costs.
- Short-circuit logic where possible to minimize operations.
Example of a Simple Token Contract
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0; contract SimpleToken { string public name = "SimpleToken"; string public symbol = "STK"; uint256 public totalSupply = 1000000; mapping(address => uint256) public balanceOf; constructor() { balanceOf[msg.sender] = totalSupply; } function transfer(address _to, uint256 _amount) public returns (bool) { require(balanceOf[msg.sender] >= _amount, "Insufficient balance"); balanceOf[msg.sender] -= _amount; balanceOf[_to] += _amount; return true; } }
Common Errors and Fixes
- Out of Gas: Make sure the contract logic is optimized.
- Reentrancy: Use checks-effects-interactions pattern and/or
reentrancyGuard
. - Integer Overflow/Underflow: Use
SafeMath
library to avoid overflows.
Key Principles
- Indentation and Spacing: Use 4 spaces for indentation and add blank lines between functions.
- Naming Conventions:
- Function names:
lowerCamelCase
- Variable names:
lowerCamelCase
- Contract names:
UpperCamelCase
- Constant variables:
UPPER_SNAKE_CASE
- Function names:
- Visibility: Always specify visibility for functions and variables (e.g.,
public
,internal
,private
). - Use of uint vs int: Prefer
uint
(unsigned integer) unless you need negative values. - Avoid Magic Numbers: Use constants instead of hardcoding numbers. Example:
uint256 constant MAX_SUPPLY = 1000000;
- Commenting: Add comments to explain complex logic or important code sections. Use
//
for inline and/* */
for block comments. - Avoiding Deprecated Features: Always use the latest stable version of Solidity.
- Gas Optimization: Use
view
orpure
functions and store values in memory or constants. - Function Modifiers: Use function modifiers for code reuse, especially for access control.
- Security Best Practices: Check for overflows/underflows and use
require
andassert
for validation.
Mastering Solidity: A Guide to Blockchain Development
Solidity has emerged as one of the most essential tools for building decentralized applications (dApps) on the Ethereum blockchain. Whether you’re a seasoned developer or new to blockchain, this guide will help you navigate the learning path and build a strong foundation in Solidity.
Understanding Solidity Basics
The first step in mastering Solidity is understanding its core components:
- Data Types: Learn about integers, strings, arrays, mappings, and custom data structures.
- Control Structures: Master loops, conditional statements, and other logic controls.
- Basic Syntax: Get familiar with how Solidity structures contracts, functions, and modifiers.
Smart Contract Development
Once the basics are in place, start creating smart contracts:
- Learn to write your first smart contract, deploy it on a testnet, and interact with it.
- Use tools like Remix IDE, Hardhat, and Truffle to streamline the development process.
- Practice creating practical contracts, like token standards (e.g., ERC-20, ERC-721).
Advanced Concepts
To stand out as a Solidity developer, explore advanced topics:
- Upgradeable Contracts: Implement proxies to make contracts upgradable.
- Design Patterns: Use patterns like factory, singleton, and more to improve code efficiency.
- Security Best Practices: Learn to protect your contracts from common vulnerabilities like reentrancy attacks.
Testing and Debugging
Quality assurance is crucial for any blockchain project:
- Write comprehensive unit tests using Mocha and Chai.
- Use tools like Hardhat or Truffle for testing and debugging.
- Simulate complex contract interactions to identify edge cases and potential bugs.
Deployment Best Practices
Deploying contracts correctly is key to a successful project:
- Use Hardhat, Truffle, or Foundry for testnet and mainnet deployments.
- Optimize gas usage to ensure cost-effective deployments.
- Securely manage private keys and deployment scripts.
Future of Solidity
The blockchain ecosystem is constantly evolving:
- Stay updated with upcoming Solidity features.
- Explore alternatives like Vyper and Rust for specialized use cases.
- Follow trends like zero-knowledge proofs and cross-chain interoperability to stay ahead.
Resources for Further Study
Here are some valuable resources to deepen your knowledge:
- Official Solidity Documentation: The go-to source for language syntax and features.
- Ethereum Developer Resources: Comprehensive tutorials and tools.
- CryptoZombies: Learn Solidity by building a gamified project.
- Solidity by Example: Experiment with practical examples.
- Udemy and Coursera: Find beginner to advanced Solidity courses.
- Ethereum StackExchange: Ask questions and get expert answers.
Create a Portfolio with Solidity Projects
Showcasing your skills is essential. Work on these project ideas:
- DeFi Protocol: Create a decentralized exchange or lending platform.
- NFT Marketplace: Build a platform for minting and trading NFTs.
- Staking DApp: Develop a staking contract with reward distribution mechanisms.
- Voting System: Design a secure, transparent decentralized voting system.
- Governance Contracts: Build contracts for DAOs to propose and vote on changes.
Deploy your projects on testnets, and share your work on GitHub to highlight your expertise.
FAQ
Q1: How long does it take to master Solidity?
If you have prior programming experience, it may take a few months to become proficient. Advanced topics and portfolio projects will require consistent practice.
Q2: What is the best way to practice Solidity?
Start with simple contracts, then work on real-world projects. Use tools like Remix, Hardhat, and Truffle to deploy contracts and gain hands-on experience.
Q3: Can I transition from Web Development to Solidity?
Yes, especially if you have JavaScript experience. Concepts like state management and event handling will be familiar, but learning blockchain principles is crucial.
Q4: Are there any certifications for Solidity developers?
While no official certifications exist, platforms like Udemy, Coursera, and blockchain bootcamps offer certificates upon course completion.
Q5: How do I stay updated with Solidity developments?
Follow Solidity’s official blog and Ethereum developer channels. Engage in online communities like StackExchange, Reddit, and Discord for the latest updates.
1 thought on “Chapter 13: Conclusion – Cheat Sheet Solidity”