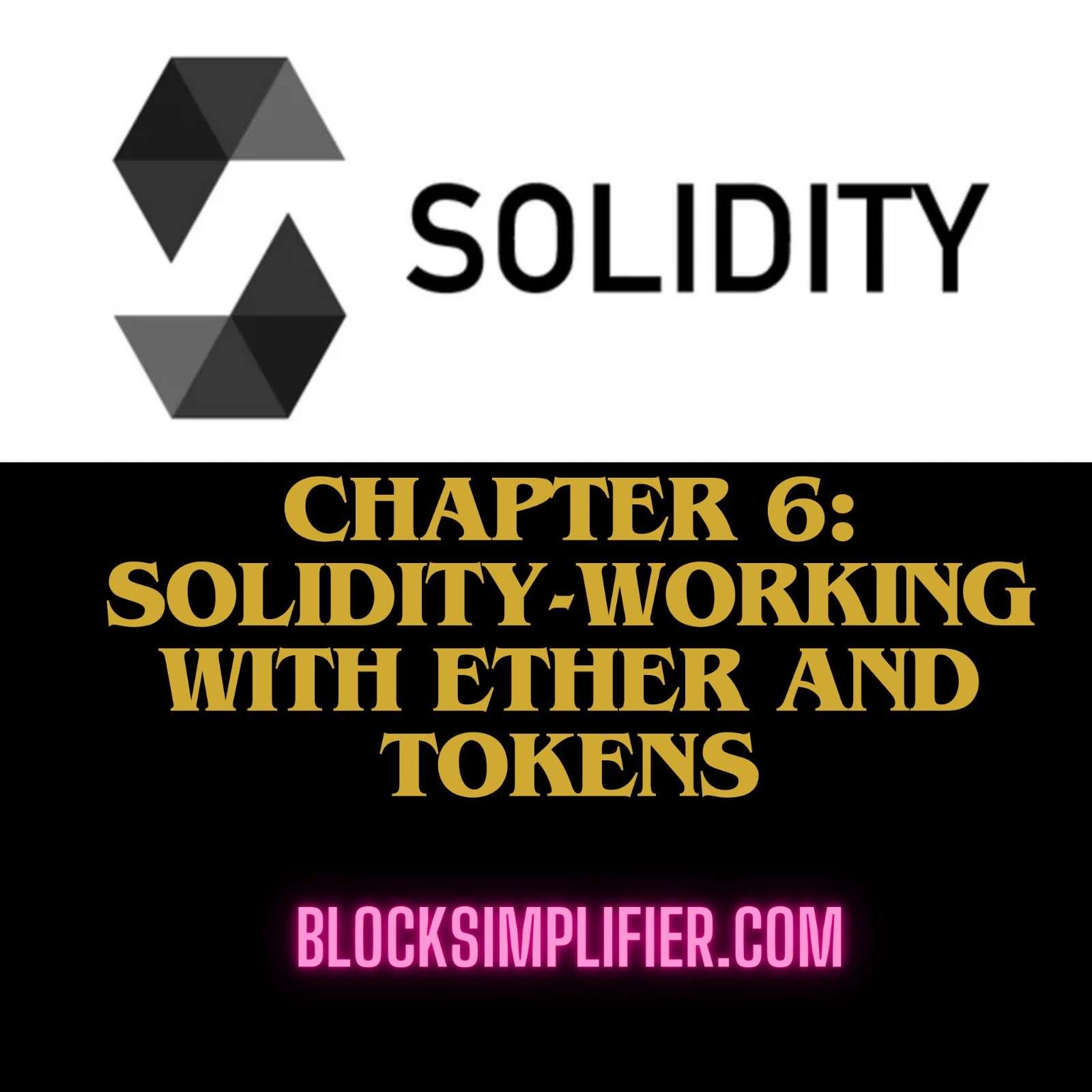
Ether (ETH) is the native cryptocurrency of the Ethereum blockchain, functioning as each a virtual asset and a application token. It is basically used to pay for transaction expenses and computational services at the Ethereum community. Unlike Bitcoin, that is designed as a decentralized forex, Ether helps Ethereum’s smart settlement functionality, permitting developers to build decentralized packages (DApps).
Ethereum employs the idea of “gas,” a measure of computational attempt required to carry out operations, which is paid in Ether. This ensures network safety and forestalls abuse by requiring customers to pay for every operation carried out on the blockchain.
Table of Contents
1. The Significance of Tokens in Blockchain Ecosystems
Tokens are virtual belongings created on blockchain structures, generally following precise requirements to make sure interoperability. They are wonderful from Ether in that they represent belongings, utilities, or rights inside a given surroundings. Tokens can be fungible (interchangeable, like ERC-20 tokens) or non-fungible (specific, like ERC-721 tokens).
Tokens play vital roles in:
- Decentralized finance (DeFi): Enabling lending, staking, and yield farming.
- Governance: Allowing token holders to take part in protocol choices.
- Gaming and NFTs: Representing in-recreation property and collectibles.
Solidity – Ether Units
In Solidity, Ether units represent different denominations of Ether. These units are useful for working with the Ethereum cryptocurrency efficiently.
Common Ether Units
Unit | Equivalent in Wei | Description |
---|---|---|
Wei | 1 Wei | Smallest denomination of Ether |
Kwei | 1,000 Wei | Thousand Wei (1e3 Wei) |
Mwei | 1,000,000 Wei | Million Wei (1e6 Wei) |
Gwei | 1,000,000,000 Wei | Billion Wei (1e9 Wei), commonly used for gas fees |
Ether | 1,000,000,000,000,000,000 Wei | 1 Ether (1e18 Wei) |
Examples
Assigning Ether Values
uint public oneEther = 1 ether; // 1 Ether = 1e18 Wei
uint public oneGwei = 1 gwei; // 1 Gwei = 1e9 Wei
uint public oneWei = 1 wei; // Smallest unit
Converting Between Units
uint public gweiToWei = 1 gwei; // 1 Gwei in Wei
uint public etherToWei = 1 ether; // 1 Ether in Wei
uint public weiToEther = 1 ether / 1e18; // Convert Wei to Ether
Sending Ether
payable(address).transfer(1 ether); // Send 1 Ether
Example Contract
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract EtherUnitsExample {
// Ether units as state variables
uint public oneEther = 1 ether;
uint public oneGwei = 1 gwei;
uint public oneWei = 1 wei;
// Convert between units
function convertToWei(uint etherAmount) public pure returns (uint) {
return etherAmount * 1 ether;
}
function convertToEther(uint weiAmount) public pure returns (uint) {
return weiAmount / 1 ether;
}
}
For further details or examples, feel free to ask!
Differences Between Ether and Tokens
Feature | Ether | Tokens |
---|---|---|
Role | Native cryptocurrency of Ethereum | Created using smart contracts |
Standard | No standard; native to Ethereum | Follow standards like ERC-20, ERC-721 |
Use Cases | Transaction fees, gas, staking | Asset representation, governance, rewards |
Creation | Issued by the Ethereum protocol | Created by developers through smart contracts |
2. Handling Ether in Smart Contracts
Basics of Smart Contract Interactions with Ether
Smart contracts are self-executing programs on the Ethereum blockchain. They can hold, send, and receive Ether, enabling functionalities like decentralized finance (DeFi) protocols, crowdfunding platforms, and escrow services. Developers often use payable
functions to accept Ether and other mechanisms for securely storing and transferring funds.
Storing and Managing Ether Securely Within Contracts
- State Variables: Contracts can use state variables to keep track of Ether balances.
- Modifiers: Use access modifiers like
onlyOwner
to restrict sensitive operations. - Reentrancy Guards: Prevent attacks by implementing security patterns such as checks-effects-interactions.
Real-World Use Cases
- Decentralized Finance (DeFi): Protocols like Uniswap use Ether for liquidity pools.
- Escrow Systems: Contracts hold Ether until predefined conditions are met.
- Payment Channels: Enable microtransactions by locking Ether temporarily.
3. Payable Functions
Explanation and Syntax of Payable Functions in Solidity
Payable functions in Solidity allow contracts to receive Ether. They are declared using the payable
keyword:
function deposit() public payable {
// Ether sent to this function will be added to the contract's balance
}
Best Practices for Receiving Ether in Contracts
- Validate Inputs: Ensure the correct amount of Ether is sent.
- Emit Events: Log transactions using events for transparency.
- Avoid Excessive Logic: Keep payable functions simple to minimize risks.
Error Handling and Fallback Mechanisms
- Fallback Function: Triggered when no other function matches the call.
- Receive Function: Introduced in Solidity 0.6.0, it handles plain Ether transfers.
receive() external payable {
// Logic to handle Ether transfers
}
fallback() external payable {
// Fallback logic
}
4. Withdrawing and Transferring Ether
Secure Withdrawal Patterns (e.g., Pull-over-Push)
The pull-over-push pattern is safer for withdrawing Ether:
- Pull: Beneficiaries call the contract to withdraw funds.
- Push: The contract actively sends funds to beneficiaries, which can be riskier.
The transfer()
, send()
, and call()
Methods: Differences and Security Considerations
Method | Gas Limit | Returns | Security Risks |
transfer() | 2300 gas | Reverts on fail | Safest but limited functionality |
send() | 2300 gas | Boolean | May fail silently |
call() | Adjustable | Boolean | Risk of reentrancy vulnerabilities |
Examples of Safe Ether Transfers
Use transfer()
or call()
with proper safeguards:
function withdraw(uint amount) public {
require(amount <= address(this).balance, "Insufficient balance");
payable(msg.sender).transfer(amount);
}
5. ERC Standards Overview
Importance of ERC Standards in the Ethereum Ecosystem
ERC (Ethereum Request for Comments) standards ensure interoperability and compatibility across the Ethereum network. They define common rules for creating tokens and other functionalities.
ERC-20: Token Fungibility and Common Use Cases
ERC-20 is the standard for fungible tokens. Key functions include:
transfer
: Moves tokens between addresses.approve
: Authorizes a spender to withdraw tokens.totalSupply
: Returns the total supply of tokens.
ERC-721: Non-Fungible Tokens (NFTs) and Their Applications
ERC-721 is used for unique tokens. Each token has a unique identifier and metadata, making it ideal for digital art and collectibles.
ERC-1155: Multi-Token Standards for Fungible and Non-Fungible Assets
ERC-1155 combines the benefits of ERC-20 and ERC-721, allowing both fungible and non-fungible tokens within a single contract. This standard is more efficient for gaming and marketplaces.
6. Creating a Basic ERC-20 Token Contract
Step-by-Step Implementation of an ERC-20 Token
- Define the Contract:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor() ERC20("MyToken", "MTK") {
_mint(msg.sender, 1000000 * 10 ** decimals());
}
}
- Deploy: Use tools like Remix or Hardhat to deploy the contract.
- Interact: Transfer tokens and check balances using tools like MetaMask.
Explanation of Key Functions and Modifiers
_mint
: Creates new tokens.decimals
: Specifies the token’s divisibility.
Deployment and Interaction Using a Test Network
Deploy on a testnet like Rinkeby or Goerli to verify functionality before mainnet deployment.
7. FAQ
Common Questions Related to Ether, Tokens, and Smart Contracts
- What is the difference between Ether and tokens?
- Ether is the native currency; tokens are assets built on Ethereum.
- How can I ensure my smart contract is secure?
- Follow best practices like reentrancy guards and access controls.
Addressing Misconceptions About Token Standards
- Misconception: ERC-20 tokens are always fungible.
- Clarification: ERC-20 defines fungibility, but specific implementations may vary.
- Misconception: NFTs are only for art.
- Clarification: NFTs have broader applications, including real estate and gaming.
8. Extra Topics
Solidity – Error Handling
Error dealing with in Solidity is a mechanism that allows builders to cope with surprising situations or disasters in smart contracts. It guarantees that the agreement reacts accurately to mistakes, preventing the execution of undesirable moves or modifications at the blockchain.
Key Concepts
- Require: Used to check if a circumstance is genuine on the begin of a feature. If the situation is fake, it throws an error and reverts the transaction.
- Assert: Similar to require but used for checking internal errors or invariants that should never fail. If it fails, it consumes all gas and reverts the transaction.
- Revert: Reverts the transaction and gives a custom blunders message, restoring the agreement state to what it was earlier than the transaction.
- Custom Errors: Developers can define their very own mistakes to deal with particular instances in a more descriptive manner.
Example of Error Handling in Solidity
// Solidity contract using error handling
pragma solidity ^0.8.0;
contract ErrorHandlingExample {
uint256 public balance;
// Require statement
function deposit(uint256 amount) public {
require(amount > 0, "Amount must be greater than zero");
balance += amount;
}
// Assert statement
function withdraw(uint256 amount) public {
assert(balance >= amount); // Ensure enough balance is available
balance -= amount;
}
// Revert statement with custom error message
function withdrawWithRevert(uint256 amount) public {
if (balance < amount) {
revert("Insufficient funds to withdraw");
}
balance -= amount;
}
}
Explanation
Require: In the deposit
function, the require
statement checks if the deposit amount is greater than zero. If it is not, the transaction is reverted, and all changes are undone.
Assert: In the withdraw
function, the assert
statement ensures the contract balance is sufficient to cover the withdrawal. If it is not, the transaction is reverted, and all gas is consumed.
Revert: In the withdrawWithRevert
function, the revert
statement is used with a custom error message to stop the transaction if there are insufficient funds.
Types of Error Handling
- require(): Checks conditions that must be true for the function to proceed. If false, it reverts the transaction with an error message.
- assert(): Used to check for conditions that should never fail (internal errors). If false, it reverts the transaction and consumes all gas.
- revert(): Reverts the transaction and restores the state, with the ability to provide a custom error message.
- Custom Errors: Developers can define custom error types to provide specific error messages for various failure scenarios.
Why Use Error Handling?
- Security: Prevents unexpected behavior and vulnerabilities by checking conditions before executing actions.
- Gas Efficiency: Reverts using
require
andrevert
ensure that gas is not wasted on failed transactions. - Readability: Custom error messages provide clearer feedback for debugging and improving contract maintenance.
Summary
- Error handling ensures that smart contracts behave as expected even in the case of errors.
- require() for checking conditions, assert() for internal invariants, and revert() for custom error handling.
- Custom errors allow more descriptive and specific error messages.
- Error handling ensures security and gas efficiency while preventing invalid operations from executing.
Solidity – Assembly
Solidity Assembly allows developers to write low-level code without delay for the Ethereum Virtual Machine (EVM). It is a way to put in writing greater green and optimized code by way of interacting directly with the EVM. Assembly in Solidity presents a more granular control over the agreement’s operations, making it feasible to get admission to and alter statistics within the maximum green way possible.
Key Points
- Assembly code is more low-level and gives you control over bytecode.
- It can be more efficient than high-level Solidity code.
- It is useful for optimizing gas costs and performing operations that are difficult or inefficient in high-level Solidity.
- Solidity provides the
assembly
keyword to write inline assembly code.
Example of Assembly in Solidity
// Solidity contract using Assembly pragma solidity ^0.8.0; contract AssemblyExample { // Adding two numbers using high-level Solidity function add(uint256 a, uint256 b) public pure returns (uint256) { return a + b; } // Adding two numbers using Assembly function addUsingAssembly(uint256 a, uint256 b) public pure returns (uint256 result) { assembly { result := add(a, b) // Assembly code to add a and b } } }
Explanation
High-Level Solidity Function (add): This is the normal way to add two numbers in Solidity, using high-level syntax.
Assembly Function (addUsingAssembly): This function uses inline assembly to perform the addition. The add()
function in assembly is a low-level function that adds two numbers. The assembly
block executes this operation and stores the result.
Why Use Assembly?
- Efficiency: Assembly allows for optimized code that can be more gas-efficient. Some operations that would normally require multiple high-level Solidity instructions can be executed in one assembly instruction.
- Low-Level Control: Assembly gives you the ability to interact directly with the EVM, providing more control over how your contract executes.
- Gas Saving: For certain tasks, using assembly can reduce gas costs because it avoids some of the overhead introduced by high-level Solidity syntax.
Real-World Example
In DeFi applications or any contract that requires heavy computation, assembly might be used to optimize critical parts of the contract where every gas saving matters. For example, in a contract that processes thousands of transactions, writing certain parts in assembly can significantly reduce the gas usage.
Summary
- Solidity Assembly: Allows you to write down low-degree code to at once engage with the Ethereum Virtual Machine (EVM).
- Efficiency and Optimization: It may be more green than high-stage Solidity code.
- Use Cases: It is useful for saving gas and handling complex operations that are difficult in high-level Solidity.
Solidity – Restricted Access
Restricted Access in Solidity is a way to control who can interact with positive functions in a clever contract. It helps defend sensitive capabilities, ensuring best legal customers can get right of entry to them. This is specifically beneficial whilst you need to allow handiest particular customers (along with the owner of the settlement or a set of directors) to execute positive actions.
Why Use Restricted Access?
Smart contracts often manage treasured assets or touchy statistics, so proscribing get admission to to sure features is vital to prevent unauthorized moves. Without limited get entry to, absolutely everyone could name the capabilities, that could result in unintentional or malicious sports.
How Restricted Access Works:
- Modifiers: Modifiers are used in Solidity to control access to functions. They are reusable code snippets that can be attached to functions to check certain conditions before the function runs.
- Owner-Only Access: The contract creator (or another specified address) is often designated as the “owner.” Only the owner can call specific functions, like transferring ownership or changing contract settings.
- Role-Based Access: Sometimes, contracts define multiple roles (like an admin or a user), where different users can perform different functions based on their assigned role.
Example of Restricted Access Using onlyOwner
Modifier:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract RestrictedAccess {
address public owner;
// Constructor to set the owner of the contract
constructor() {
owner = msg.sender; // The address that deploys the contract is the owner
}
// Modifier to check if the caller is the owner
modifier onlyOwner() {
require(msg.sender == owner, "You are not the owner!");
_;
}
// Function that can only be called by the owner
function changeOwner(address newOwner) public onlyOwner {
owner = newOwner; // Change ownership
}
// Function to check the current owner
function getOwner() public view returns (address) {
return owner;
}
}
Explanation of the Code:
- Owner Assignment: The contract’s creator is assigned as the
owner
in the constructor. This means the address that deploys the contract becomes the owner. onlyOwner
Modifier: TheonlyOwner
modifier checks if the caller is the owner. If not, it reverts the transaction with the error message “You are not the owner!”- Restricted Function: The
changeOwner
function is protected by theonlyOwner
modifier. This means only the owner can call this function and change the owner of the contract. - Check Current Owner: The
getOwner
function allows anyone to check who the current owner of the contract is.
Benefits of Restricted Access:
- Security: It ensures that only authorized users can execute critical functions (like changing the owner or withdrawing funds).
- Flexibility: You can set different levels of access for different users or roles in the contract.
- Prevention of Unauthorized Actions: It helps prevent malicious actors from accessing sensitive parts of the contract.
Solidity – Withdrawal Pattern
The Withdrawal Pattern in Solidity is a security fine exercise used to safely cope with the withdrawal of budget from a smart contract. Instead of sending finances immediately from the contract to the person, the agreement permits customers to withdraw funds themselves via calling a separate function. This pattern enables avoid capacity issues, which includes reentrancy attacks, which could occur while a agreement is sending price range directly to an outside deal with.
Why Use the Withdrawal Pattern?
When sending funds directly within a function call (e.g., during a withdrawal), the contract can become vulnerable to reentrancy attacks. This happens if the recipient address is another contract that can call back into the withdrawing function before the initial function call finishes, potentially leading to unexpected behavior or draining the contract’s funds.
The Withdrawal Pattern works by allowing users to withdraw funds at their own convenience, rather than having the contract send the funds immediately.
Steps in the Withdrawal Pattern:
1. Deposit Funds: Users send Ether to the contract.
2. Track Balances: The contract keeps track of how much each user has deposited.
3. Withdraw Funds: Users can later call a function to withdraw their balance. The contract updates the balance first, then sends the funds.
Simple Example of Withdrawal Pattern:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract WithdrawalPattern {
// Mapping to track user balances
mapping(address => uint256) public balances;
// Deposit function: Users can deposit Ether into the contract
function deposit() public payable {
balances[msg.sender] += msg.value;
}
// Withdraw function: Users can withdraw their deposited balance
function withdraw(uint256 amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance"); // Check if user has enough funds
// Update the user's balance before sending the funds
balances[msg.sender] -= amount;
// Send the amount to the user
payable(msg.sender).transfer(amount);
}
// Get contract's balance
function getContractBalance() public view returns (uint256) {
return address(this).balance;
}
}
Explanation:
1. Deposit:
– The `deposit` function allows users to send Ether to the contract. The contract records the amount sent by each user in the `balances` mapping.
2. Withdraw:
– The `withdraw` function allows users to withdraw Ether. Before the contract sends the funds, it checks that the user has enough balance.
– After checking the balance, the contract updates the user’s balance in the mapping (decreases the balance) and then transfers the funds to the user.
3. Reentrancy Safety:
– The balance is updated before the `transfer` is made. This order is important because it prevents reentrancy attacks. If the transfer were made first, a malicious contract could call the `withdraw` function again before the user’s balance is updated, potentially draining the contract’s funds.
Why This Pattern is Safe:
– No direct transfers in functions: The funds are not sent directly in the function where the balance is checked. Instead, users have to call a separate function to withdraw their funds.
– State update first: By updating the user’s balance before transferring funds, the contract avoids the risks associated with reentrancy.
2 thoughts on “Chapter 6: Solidity-Working with Ether and Tokens”